DeBox ChatWidget
DeBox ChatWidget Documentation
Overview
DeBox ChatWidget is a decentralized chat component that allows you to easily integrate real-time decentralized chat functionality into your web page. In just 10 minutes, you can embed a powerful chat program into your product, providing users with a smooth, secure, and decentralized chat experience.
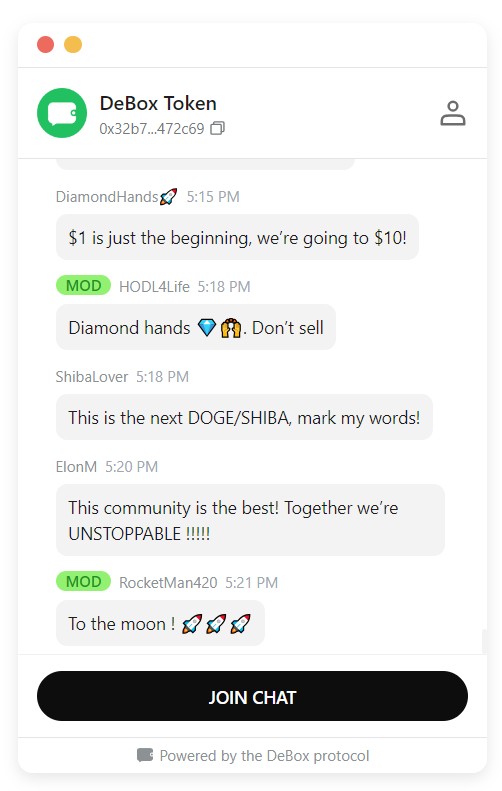
Installation and Integration
DeBox ChatWidget offers multiple integration methods, supporting flexible adaptation to different JavaScript frameworks and development environments.
Method 1: Via npm:
Suitable for projects using build tools like Webpack or Vite. Choose the appropriate ChatWidget package based on your specific framework:
-
Native HTML Project
Install the ChatWidget package for HTML projects:
npm install @debox-pro/chat-widget-html
Then import and use it in your project:
// Import in projects using build tools
import { DeBoxChatWidget } from '@debox-pro/chat-widget-html';
// Initialize ChatWidget
DeBoxChatWidget.init({
projectId: 'your-project-id',
zIndex: '999',
containerDomId: 'chat-container',
});
// Set the chat group
DeBoxChatWidget.setConversation('conversation-id'); -
React Project
Install the ChatWidget package optimized for the React framework:
npm install @debox-pro/chat-widget-react
Then import and use it in your project:
import { DeBoxChatWidget } from '@debox-pro/chat-widget-react';
function ChatComponent() {
return (
<DeBoxChatWidget
projectId="your-project-id"
conversationId="conversation-id"
onEvent={(e) => console.log('ChatWidget event:', e.detail)}
/>
);
}
Method 2: Via External Script:
Suitable for scenarios without build tools or for quick integration, by directly loading the ChatWidget UMD file through a <script>
tag.
-
Step 1: Download the UMD file
Obtain the ChatWidget UMD file from the following links and save it to your project directory:
-
Step 2: Include it in your HTML file via a
<script>
tagAssuming you saved the UMD file as index.umd.js, add the following code to your HTML file to include the ChatWidget:
<script src="./path/to/index.umd.js"></script>
<script>
// Access DeBoxChatWidget from the global object
const { DeBoxChatWidget } = window.DeBoxChatWidget;
// Initialize ChatWidget
DeBoxChatWidget.init({
projectId: 'your-project-id',
zIndex: '999',
containerDomId: 'chat-container'
});
// Set the chat group
DeBoxChatWidget.setConversation('conversation-id');
</script>Replace ./path/to/index.umd.js with the actual path to the UMD file.
Supported Methods
1. Initialize ChatWidget:
This method is used to initialize the ChatWidget plugin and should be called when the page loads.
DeBoxChatWidget.init({
projectId: getQueryVariable('xxx'),
zIndex: 'xxx',
containerDomId: 'xxx',
})
Parameters:
projectId
:string
, required, the App ID provided by the DeBox Open Platform, which needs to be applied for on the DeBox Open Platform.containerDomId
:string
, optional, specifies the container DOM element ID for the plugin, used for style isolation.zIndex
:string
, optional, default value is 999, sets the stacking order of the chat window to ensure it is not covered by other elements on the page.
2. Get Chat Group ID:
To open a chat in the ChatWidget, you need to specify the chat group ID. The chat group ID needs to be obtained through the interface provided by the DeBox Open Platform. This interface supports obtaining the corresponding chat group ID based on chain and contract address information, or group name.
DeBox Open Platform API to Get Chat Group ID
Currently, the ChatWidget floating chat plugin only supports on-chain token holding group chats. For non-on-chain token group chats, please use the DeBox website or DeBox app.
Note: This API involves your X-API-KEY
and app_secret
from the [DeBox Open Platform]. Please do not request it directly from the frontend browser. We recommend placing this request call on a private backend server.
-
Endpoint:
http://open.debox.pro/openapi/chatwidget/conversation/id
-
Request Method: POST
-
Parameters:
Request Header:
Header Type Description app_id
String Developer application identifier, which can be obtained from the [DeBox Open Platform]. X-API-KEY
String Developer API key, which can be obtained from the [DeBox Open Platform]. nonce
String Developer-generated random number, 8 characters long. timestamp
String Timestamp, accurate to milliseconds. signature
String Signature, signature
= SHA1( {app_secret
} {nonce
} {timestamp
} )- The
app_secret
used to calculate thesignature
is the developer application secret, which can be obtained from the [DeBox Open Platform].
Request Body:
Content-Type:
application/json
Parameter Name Type Required Description chain_id
Number Yes Blockchain chain ID, e.g., 1
for Ethereum,-200
for Solana.contract_address
String Yes Contract address of the token corresponding to the chat group. - The
-
Response:
- On success:
{
"code": 1,
"data": {
"cid": "xxxxxxxx"
},
"message": "success",
"success": true
}-
cid
: Chat group ID, the unique identifier of the chat group, used during initialization or when switching chat groups. -
On failure:
{
"code": 0,
"data": null,
"message": "error message",
"success": false
}
3. Switch Chat Groups:
This method is used to switch chat groups in the ChatWidget.
Note: After initializing the ChatWidget, you need to call setConversation
once to enter the chat group page:
DeBoxChatWidget.setConversation(conversationId);
Parameters:
conversationId
:string
, the ID of the chat group, which can be obtained through the DeBox Open Platform API. See above for details.
4. Listen to ChatWidget Events:
This method is used to listen to events from the ChatWidget. Developers can handle events as needed.
DeBoxChatWidget.addEventListener(e => {
// handle events
});
5. Destroy ChatWidget:
This method is used to destroy the ChatWidget element.
DeBoxChatWidget.destroy();
Integration Example
We provide a simple development example for reference, allowing developers to quickly integrate the ChatWidget into their own projects.
Online Demo Links | Source Code Links |
---|---|
[html-umd Integration Example] | [Source Code] |
[html-npm Integration Example] | [Source Code] |
[react-npm Integration Example] | [Source Code] |
Native HTML Integration Example:
0. Online Demo:
1. Import the ChatWidget Plugin:
In this example, the ChatWidget plugin is imported using the UMD file:
<script src="./ChatWidget.umd.js"></script>
2. Initialize the ChatWidget Plugin:
In this example, the DeBox ChatWidget is initialized when the web page loads.
<script>
window.DeBoxChatWidget.init({
projectId: getQueryVariable('projectId'),
zIndex: '9999',
containerDomId: 'test-dom',
});
</script>
3. Retrieve the Chat Group ID for the Page:
Developers can call the DeBox Open Platform API to get the chat group ID corresponding to a specific token on a webpage (see above for details).
In this example, the ChatWidget uses the chat group corresponding to the information in the URL by default when it opens. During page initialization, it uses the token information and group information from the URL to call a private backend interface that encapsulates the DeBox Open Platform service to get the chat group ID corresponding to the token:
Code Example:
<script>
// Check for input parameters in the URL and initialize Conversation ID if present
async function initializeConversationId() {
const groupName = getQueryVariable('group_name');
const chainId = getQueryVariable('chain_id');
const contractAddress = getQueryVariable('contract_address');
if (groupName || chainId || contractAddress) {
const groupParam = groupName ? encodeURIComponent(groupName) : '';
const chainParam = chainId ? encodeURIComponent(chainId) : '';
const addressParam = contractAddress ? encodeURIComponent(contractAddress) : '';
// use your own backend endpoint:
const url = `https://xxxx.xxx/getconversationid?group_name=${groupParam}&chain_id=${chainParam}&contract_address=${addressParam}`;
try {
const response = await fetch(url);
const data = await response.json();
if (data.success) {
const conversationId = data.data.cid;
DeBoxChatWidget.setConversation(conversationId);
} else {
console.warn("Failed to retrieve Conversation ID during initialization");
}
} catch (error) {
console.error("Error fetching Conversation ID during initialization", error);
}
}
}
</script>
This example also provides an entry point for manually obtaining chat group information. You can use the target token information and group information entered on the web page to obtain the chat group ID and switch to the corresponding chat group when needed:
Code Example:
<h3>Get Conversation ID using given parameters:</h3>
<div>
<input type="text" id="groupName" placeholder="Group Name" />
<input type="text" id="chainId" placeholder="Chain ID" />
<input type="text" id="contractAddress" placeholder="Contract Address" />
<button onclick="getConversationId()">Get Conversation ID</button>
</div>
<script>
async function getConversationId() {
const groupName = document.getElementById('groupName').value;
const chainId = document.getElementById('chainId').value;
const contractAddress = document.getElementById('contractAddress').value;
// use your own backend endpoint:
const url = `https://xxxx.xxx/getconversationid?group_name=${groupParam}&chain_id=${chainParam}&contract_address=${addressParam}`;
if (!groupParam && !chainParam && !addressParam) {
alert("Please fill in at least one field.");
return;
}
try {
const response = await fetch(url);
const data = await response.json();
if (data.success) {
const conversationId = data.data.cid;
document.getElementById('conversationInput').value = conversationId;
alert(`Conversation ID: ${conversationId}`);
} else {
alert("Failed to get conversation ID");
}
} catch (error) {
alert("Error fetching conversation ID");
console.error(error);
}
}
</script>
-
Note: Since the API call to get the chat group ID involves the
X-API-KEY
andapp_secret
from the [DeBox Open Platform], it is recommended that developers place the implementation of this API call on a private backend server. -
In this example, the developer places the use of sensitive information such as
X-API-KEY
andapp_secret
on a private backend server and creates a private interface for the frontend to call, ensuring that private information is not exposed.
Server-side private interface code example:
package main
import (
"crypto/sha1"
"encoding/hex"
"encoding/json"
"fmt"
"io"
"math/rand"
"net/http"
"os"
"strconv"
"strings"
"time"
"github.com/gin-gonic/gin"
"github.com/joho/godotenv"
"github.com/redis/go-redis/v9"
"golang.org/x/net/context"
)
var (
rdb *redis.Client
)
func initializeRedis() *redis.Client {
redisAddr := os.Getenv("REDIS_ADDR")
redisPassword := os.Getenv("REDIS_PASSWORD")
redisDB, err := strconv.Atoi(os.Getenv("REDIS_DB"))
if err != nil {
fmt.Println("Invalid REDIS_DB value in .env file:", err)
return nil
}
rdb = redis.NewClient(&redis.Options{
Addr: redisAddr,
Password: redisPassword,
DB: redisDB,
})
// Check the redis connection
err = rdb.Ping(context.Background()).Err()
if err != nil {
fmt.Println("Redis connection failed:", err)
return nil
}
return rdb
}
func calculateSignature(appSecret, nonce, timestamp string) string {
h := sha1.New()
h.Write([]byte(appSecret + nonce + timestamp))
return hex.EncodeToString(h.Sum(nil))
}
func main() {
// Load environment variables from .env.local file
if err := godotenv.Load(".env.local"); err != nil {
fmt.Println("Error loading .env file")
}
appSecret := os.Getenv("APP_SECRET")
apiKey := os.Getenv("API_KEY")
appId := os.Getenv("APP_ID")
port := os.Getenv("PORT")
redisExpiryTime := os.Getenv("REDIS_EXPIRY_TIME")
redisExpiryDuration, err := time.ParseDuration(redisExpiryTime)
if err != nil {
fmt.Println("Invalid REDIS_EXPIRY_TIME value in .env file:", err)
return
}
// Initialize Redis
rdb := initializeRedis()
if rdb == nil {
fmt.Println("Redis initialization failed, exiting")
return
}
fmt.Println("Redis initialized")
gin.SetMode(gin.ReleaseMode)
r := gin.Default()
// Define a route to get conversation ID
r.GET("/getconversationid", func(c *gin.Context) {
ctx := c.Request.Context()
// Get parameters from the query string
groupName := c.Query("group_name")
chainID := c.Query("chain_id")
contractAddress := c.Query("contract_address")
if groupName == "" && chainID == "" && contractAddress == "" {
c.JSON(http.StatusBadRequest, gin.H{"error": "Missing required parameters", "success": false})
return
}
// Set request URL
url := "http://open.debox.pro/openapi/chatwidget/conversation/id"
method := "POST"
nonce := fmt.Sprintf("%08d", rand.Intn(100000000))
timestamp := fmt.Sprintf("%d", time.Now().UnixMilli())
signature := calculateSignature(appSecret, nonce, timestamp)
// Construct request payload
payloadMap := make(map[string]interface{})
if groupName != "" {
payloadMap["group_name"] = groupName
}
if chainID != "" {
chainIDInt, err := strconv.Atoi(chainID)
if err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": "Invalid chain_id format", "success": false})
return
}
payloadMap["chain_id"] = chainIDInt
}
if contractAddress != "" {
payloadMap["contract_address"] = contractAddress
}
// Convert payload to JSON
payloadBytes, err := json.Marshal(payloadMap)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to construct request payload", "success": false})
return
}
// Redis cache key
cacheKey := fmt.Sprintf("conversation:%s:%s:%s", groupName, chainID, contractAddress)
// Check if the response is already cached
cachedResponse, err := rdb.Get(ctx, cacheKey).Result()
if err == nil {
// Cache hit, return cached data
c.Data(http.StatusOK, "application/json", []byte(cachedResponse))
return
} else if err != redis.Nil {
// Redis error
c.JSON(http.StatusInternalServerError, gin.H{"error": "server Redis error", "success": false})
return
}
// Make the request to Debox Open API
client := &http.Client{}
req, err := http.NewRequest(method, url, strings.NewReader(string(payloadBytes)))
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()})
return
}
// Add headers
req.Header.Add("app_id", appId)
req.Header.Add("X-API-KEY", apiKey)
req.Header.Add("nonce", nonce)
req.Header.Add("timestamp", timestamp)
req.Header.Add("signature", signature)
req.Header.Add("Content-Type", "application/json")
// Send request
res, err := client.Do(req)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()})
return
}
defer res.Body.Close()
body, err := io.ReadAll(res.Body)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()})
return
}
// Cache the response for future use
err = rdb.Set(ctx, cacheKey, body, redisExpiryDuration).Err()
if err != nil {
fmt.Println("Failed to cache the response:", err)
}
c.Data(res.StatusCode, "application/json", body)
})
// Start the server
fmt.Println("Server is running on port " + port)
r.Run(":" + port)
}
4. Initialize Chat:
After obtaining the chat group ID, you need to manually call the setConversation
function to enter the chat group page:
<script>
window.DeBoxChatWidget.setConversation('1f2IELs0');
</script>
5. Integration Complete, Users Can Start Chatting:
After successfully initializing the chat widget, users can view the chat of token holders in the corresponding token chat group.
Users can view a certain number of messages in the chat group without connecting their wallet. If users want to speak in the group, they need to connect the plugin with a wallet holding the token.
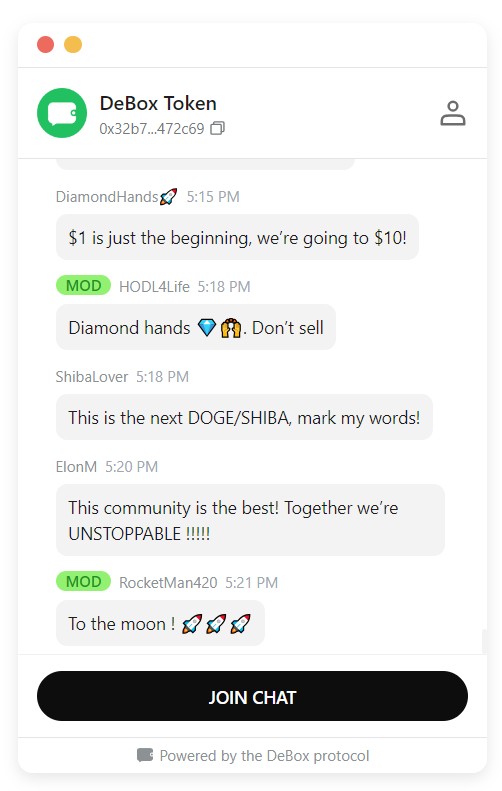
6. Switch Chat Groups:
In this example, users can switch to the corresponding chat group by entering the target chat group ID.
In actual development, developers should obtain the corresponding token chat group ID as needed and switch the ChatWidget to the corresponding chat group.
<script>
function setConversationFromInput() {
const inputValue = document.getElementById('conversationInput').value;
window.DeBoxChatWidget.setConversation(inputValue);
}
</script>
7. Listen to Plugin Events:
In this example, events from the Debox ChatWidget are listened to and output.
During actual development, developers can listen to events and handle them as needed.
<script>
window.DeBoxChatWidget.addEventListener(e => {
const events = document.getElementById('events');
const li = document.createElement('li');
li.innerHTML = JSON.stringify(e.detail);
events.appendChild(li);
});
</script>
8. Destroy the Component:
In this example, a button is provided to destroy the ChatWidget. Users can click the button to destroy the ChatWidget.
<script>
window.DeBoxChatWidget.destroy();
</script>
Complete Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>HTML UMD Example</title>
<meta name="viewport"
content="viewport-fit=cover, width=device-width, initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no" />
</head>
<body style="margin: 0">
<div id="app" style="min-height: 100vh; width: 100vw; word-wrap: break-word">
<main>
<!-- Plugin load position -->
<div id="test-dom">
<h2>This is the dApp page...</h2>
</div>
<br />
<h3>Retrieve Conversation ID based on input parameters:</h3>
<div>
<input type="text" id="groupName" placeholder="Group Name" />
<input type="text" id="chainId" placeholder="Chain ID" />
<input type="text" id="contractAddress" placeholder="Contract Address" />
<button onclick="getConversationId()">Get Conversation ID</button>
</div>
<h3>Input Conversation ID to change the chat:</h3>
<div>
<input type="text" id="conversationInput" placeholder="Enter conversation ID here" />
<button onclick="setConversationFromInput()">Change Conversation</button>
</div>
<h3>Destroy the plugin:</h3>
<button onclick="window.DeBoxChatWidget.destroy()">Destroy</button>
<br />
<h3>Event Log:</h3>
<div id="events"></div>
</main>
<br />
</div>
<script src="./index.umd.js"></script>
<script>
const { DeBoxChatWidget } = window.DeBoxChatWidget;
function getQueryVariable(variable) {
return new URL(window.location.href).searchParams.get(variable);
}
// Initialize plugin
DeBoxChatWidget.init({
projectId: getQueryVariable('project_id'),
zIndex: '9999',
containerDomId: 'test-dom',
});
// Check for input parameters in the URL and initialize Conversation ID if present
async function initializeConversationId() {
const groupName = getQueryVariable('group_name');
const chainId = getQueryVariable('chain_id');
const contractAddress = getQueryVariable('contract_address');
if (groupName || chainId || contractAddress) {
const groupParam = groupName ? encodeURIComponent(groupName) : '';
const chainParam = chainId ? encodeURIComponent(chainId) : '';
const addressParam = contractAddress ? encodeURIComponent(contractAddress) : '';
// use your own backend endpoint:
const url = `https://xxxx.xxx/getconversationid?group_name=${groupParam}&chain_id=${chainParam}&contract_address=${addressParam}`;
try {
const response = await fetch(url);
const data = await response.json();
if (data.success) {
const conversationId = data.data.cid;
DeBoxChatWidget.setConversation(conversationId);
} else {
console.warn("Failed to retrieve Conversation ID during initialization");
}
} catch (error) {
console.error("Error fetching Conversation ID during initialization", error);
}
}
}
// Execute initialization logic
initializeConversationId();
// Change the conversation
function setConversationFromInput() {
const inputValue = document.getElementById('conversationInput').value;
if (inputValue) {
DeBoxChatWidget.setConversation(inputValue);
} else {
alert('Please enter a valid Conversation ID');
}
}
// Fetch Conversation ID based on inputs
async function getConversationId() {
const groupName = document.getElementById('groupName').value;
const chainId = document.getElementById('chainId').value;
const contractAddress = document.getElementById('contractAddress').value;
const groupParam = groupName ? encodeURIComponent(groupName) : '';
const chainParam = chainId ? encodeURIComponent(chainId) : '';
const addressParam = contractAddress ? encodeURIComponent(contractAddress) : '';
if (!groupParam && !chainParam && !addressParam) {
alert("Please fill in at least one field.");
return;
}
const url = `https://intis.top/getconversationid?group_name=${groupParam}&chain_id=${chainParam}&contract_address=${addressParam}`;
try {
const response = await fetch(url);
const data = await response.json();
if (data.success) {
const conversationId = data.data.cid;
document.getElementById('conversationInput').value = conversationId;
alert(`Conversation ID: ${conversationId}`);
} else {
alert("Failed to get Conversation ID");
}
} catch (error) {
alert("Error fetching Conversation ID");
console.error(error);
}
}
// Listen for plugin events
DeBoxChatWidget.addEventListener((e) => {
const events = document.getElementById('events');
const li = document.createElement('li');
li.innerHTML = JSON.stringify(e.detail);
events.appendChild(li);
});
</script>
</body>
</html>